Programming in C Data Structure
Summary:
This note provides a collection of data structures and algorithms implemented in Programming in C Data Structure. The list includes stack, queue, circular queue, linked list, and sorting algorithms such as insertion sort, selection sort, bubble sort, and quick sort. It also includes search algorithms like sequential search, binary search, and binary search trees.
Excerpt:
Programming in C Data Structure
Stack using Array
#include<stdio.h>
#include<stdlib.h>
# define s 5
int top=-1,stk[s];
void main (){
int ch=0;
while(ch<4){system(“cls”);
printf(“\n\t1. Push\n\t2. Pop\n\t3. List\n\t4. Exit\n\n\t enter your choice:”);
scanf(“%d”,&ch);
switch(ch){
case 1:
push();
break;
case 2 :
pop();
break;
case 3:
list();
break;
}
}
}
push(){
int a;
if(top==s-1){
printf(“\n\n\t stack is overflow”);
getch();
return;
}
printf(“\n\n\t enter data to push”);
scanf(“%d”,&a);
top++;
stk[top]=a;
printf(“\n\tdata is pushed”);
getch();
}
pop(){
if(top==-1){
printf(“\n\tstack is underflow”);
getch();
return;
}
printf(“\n\n\tpoped data is:%d”,stk[top]);
top–;
getch();
}
list(){
int i;
if(top==-1){
printf(“\n\n\tstack is underflow”);
getch();
return;
}
printf(“\n\n”);
for(i=top;i>=0;i–);
printf(“\n\t%d”,stk[i]);
getch();
}
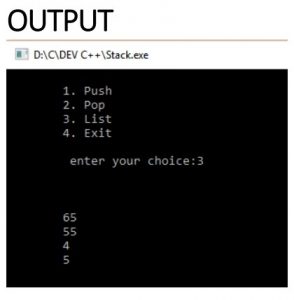
Programming in C Data Structure
Queue using array
#include<stdio.h>
#include<stdlib.h>
#define S 5
void insert();
void removes();
void display();
int q[S], R=-1, F=-1;
int main() {
int ch=0;
while(ch<4) {
system(“cls”);
printf(“Input the choice\n\t1.Insert\n\t2.Remove\n\t3.Display\n\t4.Exit\n”);
ch=getche()-‘0’;
switch(ch) {
case 1: insert();
getch();
break;
case 2: removes();
getch();
break;
case 3: display();
getch();
break;
}
}
return 0;
}
void insert() {
if(R==S-1) {
printf(“\n\tQueue is full\n”);
return;
}
printf(“\nEnter the element : “);
scanf(“%d”, &q[++R]);
if(F==-1)
++F;
display();
}
void removes() {
if((R==-1)) {
printf(“\n\tQueue empty\n”);
return;
}
printf(“\nElement removed = %d”, q[F++]);
if(F==R+1)
F=R=-1;
display();
}
void display() {
int i;
if(F==-1||(F==R+1)) {
printf(“\n\tQueue is empty\n”);
return;
}
printf(“\n\t”);
for(i=F;i<=R;i++)
printf(“%d “, q[i]);
printf(“\n”);
}
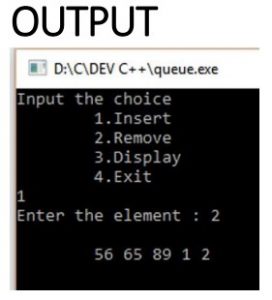
Programming in C Data Structure
Circular queue using array
#include<stdio.h>
#include<stdlib.h>
#define S 5
void insert();
void removes();
void display();
int Cq[S], R=-1, F=-1;
int main() {
int ch=0;
while(ch<4) {
system(“cls”);
printf(“Input the choice\n\t1.Insert\n\t2.Remove\n\t3.Display\n\t4.Exit\n”);
ch=getche()-‘0’;
switch(ch) {
case 1: insert();
getch();
break;
case 2: removes();
getch();
break;
case 3: display();
getch();
break;
}
}
return 0;
}
void insert() {
if((R==S-1&&F==0)||R==F-1) {
printf(“\n\tQueue is full\n”);
return;
}
printf(“\nEnter the element : “);
if(R==S-1)
R=-1;
else if(F==-1)
++F;
scanf(“%d”, &Cq[++R]);
display();
}
void removes() {
if(R==-1) {
printf(“\n\tQueue empty\n”);
return;
}
printf(“\nElement removed = %d”, Cq[F++]);
if(F==R+1)
F=R=-1;
else if(F==S)
F=0;
display();
}
void display() {
int i;
if(F==-1) {
printf(“\n\tQueue is empty\n”);
return;
}
printf(“\n\t”);
if(R<F) {
for(i=0;i<=R;i++)
printf(“%d “, Cq[i]);
for(i=R+1;i<F;i++)
printf(” “);
for(i=F;i<S;i++)
printf(“%d “, Cq[i]);
printf(“\n”);
return;
}
for(i=F;i<=R;i++)
printf(“%d “, Cq[i]);
printf(“\n”);
}
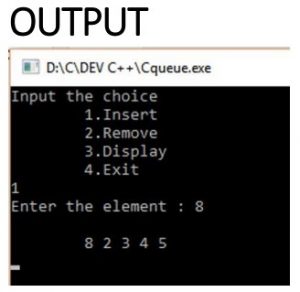
Programming in C Data Structure
Reviews